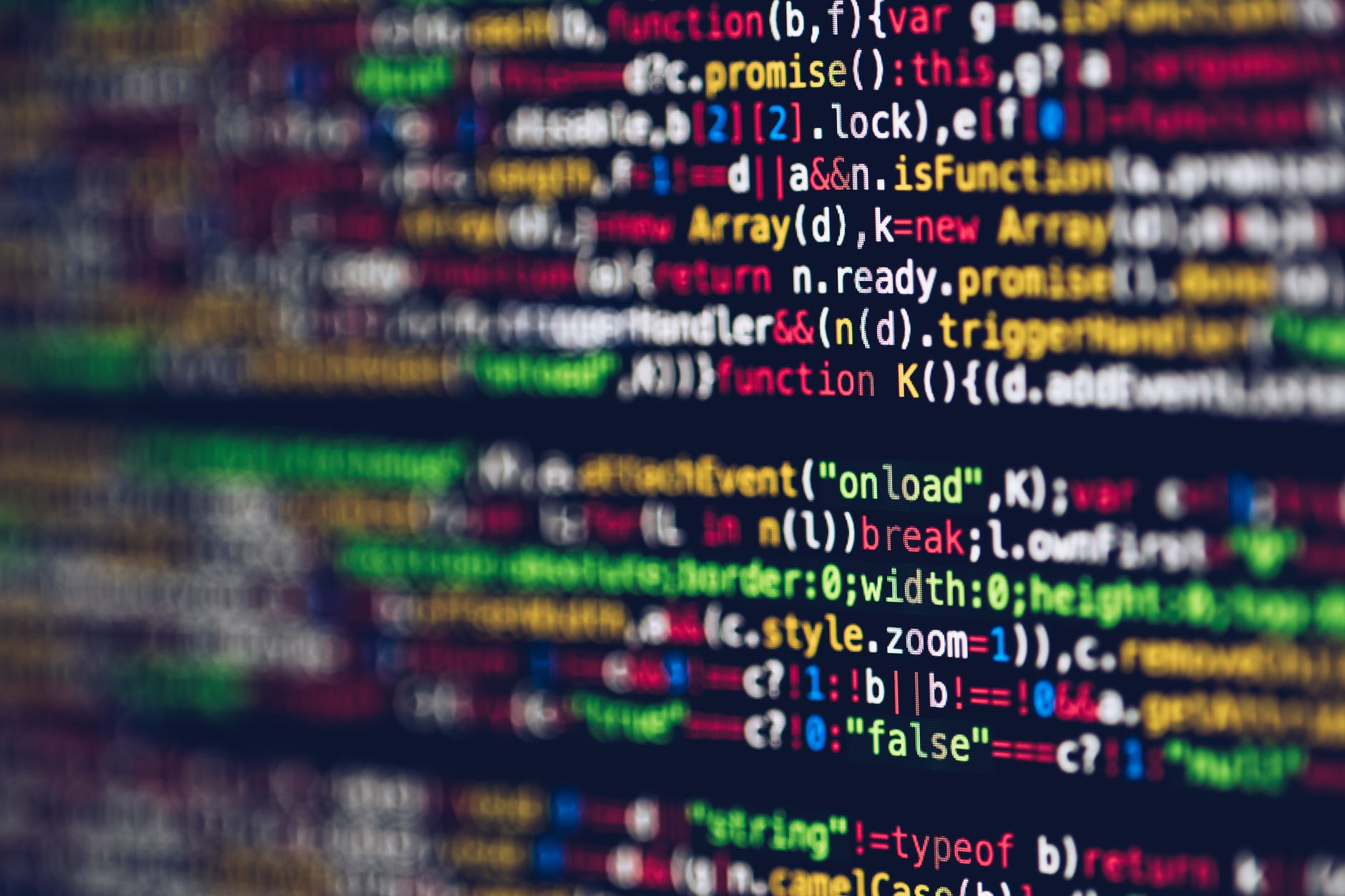
The construction of a POST request in Angular is simple. But I’ve probably searched too many times on how to do it because I can memorize the syntax. It’s time to put down some notes. I’m going to go through how to add a header, create a body and add parameters to a POST request in Angular.
1. Inject HttpClient
We need the HttpClient to perform a POST request in Angular. It handles a lot of things for you so you don’t have to reinvent the wheel. To do this, simply add a private parameter in the constructor of your component or service:
constructor(private http: HttpClient)
2. Create HttpHeaders
You can simply create an HttpHeaders object. Then append header names and values to it. For example:
const headers = new HttpHeaders()
.append(
'Content-Type',
'application/json'
);
3. Create Body
Technically, you can send any data type in the body. But most of the time I would convert an object to a JSON string. You can specify the content-type in the headers.
const body=JSON.stringify(myObject);
4. Create HttpParams
You can make a POST request with multiple parameters. To do that, create a new HttpParams object and append the desired parameters to it.
const params = new HttpParams()
.append('param1', 'some data 1')
.append('param2', 'some data 2');
5. Make a POST request
After building the headers, body, and parameters, you can make a POST request with the following code. You don’t have to provide all the things above. For example, if your API doesn’t care about the body, you can leave it as an empty string.
this.http
.post<any>('https://api-example.com', body, {
headers: headers,
params: params,
})
.subscribe((res) => console.log(res));
That’s it. Happy coding! 🙂
If my note helped, please consider buying me a coffee😁.
Lok